Before you begin: Join our book community on Discord
Give your feedback straight to the author himself and chat to other early readers on our Discord server (find the “architecting-aspnet-core-apps-3e” channel under EARLY ACCESS SUBSCRIPTION).
https://packt.link/EarlyAccess
This chapter covers the building blocks of the next chapter, which is about Vertical Slice Architecture. We begin with a quick overview of Vertical Slice Architecture to give you an idea of the end goal. Then, we explore the Mediator design pattern, which plays the role of the middleman between the components of our application. That leads us to the Command Query Responsibility Segregation (CQRS) pattern, which describes how to divide our logic into commands and queries. Finally, we consolidate our learning by exploring MediatR, an open-source implementation of the Mediator design pattern, and send queries and commands through it to demonstrate how the concepts we have studied so far come to life in real-world application development.In this chapter, we cover the following topics:
- A high-level overview of Vertical Slice Architecture
- Implementing the Mediator pattern
- Implementing the CQS pattern
- Code smell – Marker Interfaces
- Using MediatR as a mediator
Let’s begin with the end goal.
A high-level overview of Vertical Slice Architecture
Before starting, let’s look at the end goal of this chapter and the next. This way, it should be easier to follow the progress toward that goal throughout the chapter.As we covered in Chapter 14, Layering and Clean Architecture, a layer groups classes together based on shared responsibilities. So, classes containing data access code are part of the data access layer (or infrastructure). People represent layers using horizontal slices in diagrams like this:
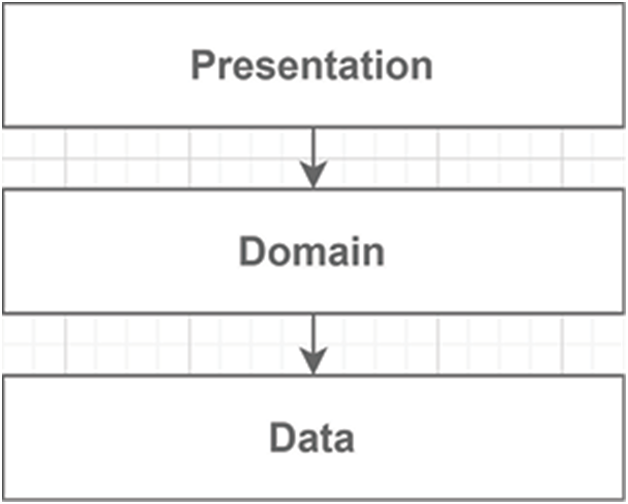
Figure 16.1: Diagram representing layers as horizontal slices
The “vertical slice” in “Vertical Slice Architecture” comes from that; a vertical slice represents the part of each layer that creates a specific feature. So, instead of dividing the application into layers, we divide it into features. A feature manages its data access code, domain logic, and possibly even presentation code. The key is to loosely couple the features from one another and keep each feature’s components close together. In a layered application, when we add, update, or remove a feature, we must change one or more layers, which too often translates to “all layers.”On the other hand, with vertical slices, we keep features isolated, allowing us to design them independently. From a layering perspective, this is like flipping your way of thinking about software to a 90° angle:
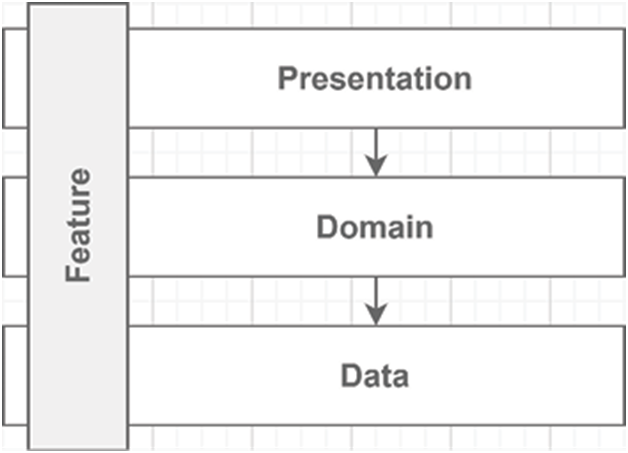
Figure 16.2: Diagram representing a vertical slice crossing all layers
Vertical Slice Architecture does not dictate the use of CQRS, the Mediator pattern, or MediatR, but these tools and patterns flow very well together, as we see in the next chapter. Nonetheless, these are just tools and patterns that you can use or change in your implementation using different techniques; it does not matter and does not change the concept.
We explore additional ways of building feature-oriented applications in Chapter 18, Request-EndPoint-Response (REPR), and Chapter 20, Modular Monolith.
The goal is to encapsulate features together, use CQRS to divide the application into requests (commands and queries), and use MediatR as the mediator of that CQRS pipeline, decoupling the pieces from one another.You now know the plan. We explore Vertical Slice Architecture later. Meanwhile, let’s begin with the Mediator design pattern.
Leave a Reply